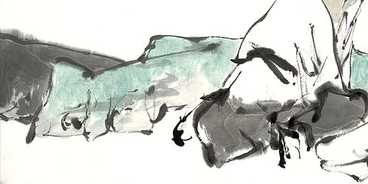
1、本地安装
$ npm install eslint --save-dev
生成配置文件
$ ./node_modules/.bin/eslint --init
之后,您可以运行ESLint在任何文件或目录如下:
$ ./node_modules/.bin/eslint index.js
index.js是你需要测试的js文件。你使用的任何插件或共享配置(必须安装在本地来与安装在本地的ESLint一起工作)。
2、全局安装
如果你想让ESLint可用到所有的项目,建议全局安装ESLint。
$ npm install -g eslint
生成配置文件
$ eslint --init
之后,您可以在任何文件或目录运行ESLint
$ eslint index.js
PS:eslint –init是用于每一个项目设置和配置eslint,并将执行本地安装的ESLint及其插件的目录。如果你喜欢使用全局安装的ESLint,在你配置中使用的任何插件都必须是全局安装的。
使用
1、在项目根目录生成package.json文件(配置ESLint的项目中必须有 package.json 文件,如果没有的话可以使用 npm init -y来生成)
$ npm init -y
2、安装eslint(安装方式根据个人项目需要安装,这里使用全局安装)
$ npm install -g eslint
3、创建index.js文件,里面写一个函数。
function merge () { var ret = {}; for (var i in arguments) { var m = arguments[i]; for (var j in m) ret[j] = m[j]; } return ret;}console.log(merge({a: 123}, {b: 456}));
执行node index.js,输出结果为{ a: 123, b: 456 }
$ node index.js{ a: 123, b: 456 }
使用eslint检查
$ eslint index.js
Oops! Something went wrong! :(ESLint: 4.19.1.ESLint couldn't find a configuration file. To set up a configuration file for this project, please run: eslint --initESLint looked for configuration files in E:\website\demo5\js and its ancestors. If it found none, it then looked in your home directory.If you think you already have a configuration file or if you need more help, please stop by the ESLint chat room: https://gitter.im/eslint/eslint
执行结果是失败,因为没有找到相应的配置文件,个人认为这个eslint最重要的就是配置问题。
新建配置文件
$ eslint --init
生成的过程中,需要选择生成规则、支持环境等内容,下面说明一些本人的生成选项
? How would you like to configure ESLint? Answer questions about your style? Are you using ECMAScript 6 features? Yes? Are you using ES6 modules? Yes? Where will your code run? Browser? Do you use CommonJS? Yes? Do you use JSX? No? What style of indentation do you use? Tabs? What quotes do you use for strings? Single? What line endings do you use? Windows? Do you require semicolons? No? What format do you want your config file to be in? JavaScript
生成的内容在.eslintrc.js文件中,文件内容如下
module.exports = { "env": { "browser": true, "commonjs": true, "es6": true }, "extends": "eslint:recommended", "parserOptions": { "sourceType": "module" }, "rules": { "indent": [ "error", "tab" ], "linebreak-style": [ "error", "windows" ], "quotes": [ "error", "single" ], "semi": [ "error", "never" ] }};
不过这个生成的额文件里面已经有一些配置了,把里面的内容大部分删除。留下个extends,剩下的自己填就可以了
module.exports = { "extends": "eslint:recommended" };
eslint:recommended配置,它包含了一系列核心规则,能报告一些常见的问题。
重新执行eslint index.js,输出如下
10:1 error Unexpected console statement no-console 10:1 error 'console' is not defined no-undef✖ 2 problems (2 errors, 0 warnings)
Unexpected console statement no-console — 不能使用console
‘console’ is not defined no-undef — console变量未定义,不能使用未定义的变量
一条一条解决,不能使用console的提示,那我们就禁用no-console就好了,在配置文件中添加rules
module.exports = { extends: 'eslint:recommended', rules: { 'no-console': 'off', },};
配置规则写在rules对象里面,key表示规则名称,value表示规则的配置。
然后就是解决no-undef:出错的原因是因为JavaScript有很多种运行环境,比如常见的有浏览器和Node.js,另外还有很多软件系统使用JavaScript作为其脚本引擎,比如PostgreSQL就支持使用JavaScript来编写存储引擎,而这些运行环境可能并不存在console这个对象。另外在浏览器环境下会有window对象,而Node.js下没有;在Node.js下会有process对象,而浏览器环境下没有。
所以在配置文件中我们还需要指定程序的目标环境:
module.exports = { extends: 'eslint:recommended', env: { node: true, }, rules: { 'no-console': 'off', }};
再重新执行检查时,就没有任何提示输出了,说明index.js已经完全通过了检查。
配置
配置方式有两种:文件配置方式、代码注释配置方式(建议使用文件配置的形式,比较独立,便于维护)。
使用文件配置的方式:在项目的根目录下,新建一个名为 .eslintrc 的文件,在此文件中添加一些检查规则。
文件配置方式
env:你的脚本将要运行在什么环境中
Environment可以预设好的其他环境的全局变量,如brower、node环境变量、es6环境变量、mocha环境变量等
'env': { 'browser': true, 'commonjs': true, 'es6': true},
globals:额外的全局变量
globals: { vue: true, wx: true},
rules:开启规则和发生错误时报告的等级
规则的错误等级有三种:
0或’off’:关闭规则。 1或’warn’:打开规则,并且作为一个警告(并不会导致检查不通过)。 2或’error’:打开规则,并且作为一个错误 (退出码为1,检查不通过)。参数说明: 参数1 : 错误等级 参数2 : 处理方式
配置代码注释方式
使用 JavaScript 注释把配置信息直接嵌入到一个文件
示例:
忽略 no-undef 检查 /* eslint-disable no-undef */忽略 no-new 检查 /* eslint-disable no-new */设置检查 /*eslint eqeqeq: off*/ /*eslint eqeqeq: 0*/
配置和规则的内容有不少,有兴趣的同学可以参考这里:rules
使用共享的配置文件
我们使用配置js文件是以extends: ‘eslint:recommended’为基础配置,但是大多数时候我们需要制定很多规则,在一个文件中写入会变得很臃肿,管理起来会很麻烦。
新建一个文件比如eslint-config-public.js,在文件内容添加一两个规则。
module.exports = { extends: 'eslint:recommended', env: { node: true, }, rules: { 'no-console': 'off', 'indent': [ 'error', 4 ], 'quotes': [ 'error', 'single' ], },};
然后原来的.eslintrc.js文件内容稍微变化下,删掉所有的配置,留下一个extends。
module.exports = { extends: './eslint-config-public.js',};
这个要测试的是啥呢,就是看看限定缩进是4个空格和使用单引号的字符串等,然后测试下,运行eslint index.js,得到的结果是没有问题的,但是如果在index.js中的var ret = {};前面加个空格啥的,结果就立马不一样了。
2:1 error Expected indentation of 4 spaces but found 5 indent✖ 1 problem (1 error, 0 warnings) 1 error, 0 warnings potentially fixable with the `--fix` option.
这时候提示第2行的是缩进应该是4个空格,而文件的第2行却发现了5个空格,说明公共配置文件eslint-config-public.js已经生效了。
除了这些基本的配置以外,在npm上有很多已经发布的ESLint配置,也可以通过安装使用。配置名字一般都是eslint-config-为前缀,一般我们用的eslint是全局安装的,那用的eslint-config-模块也必须是全局安装,不然没法载入。
在执行eslint检查的时候,我们会经常看到提示“–flx”选项,在执行eslint检查的时候添加该选项会自动修复部分报错部分(注意这里只是部分,并不是全部)
比如我们在规则中添加一条no-extra-semi: 禁止不必要的分号。
'no-extra-semi':'error'
然后,我们在index.js最后多添加一个分号
function merge () { var ret = {}; for (var i in arguments) { var m = arguments[i]; for (var j in m) ret[j] = m[j]; } return ret;;}console.log(merge({a: 123}, {b: 456}));
执行eslint index.js,得到结果如下:
7:16 error Unnecessary semicolon no-extra-semi 7:16 error Unreachable code no-unreachable✖ 2 problems (2 errors, 0 warnings) 1 error, 0 warnings potentially fixable with the `--fix` option.
然后我们在执行eslint index.js –fix就会自动修复,index.js那个多余的分号也就被修复消失不见了。
总结
以上是我在学习eslint整理的一些资料,不算太全面,对于像我这样的新手入门足够了